Mastering React: Essential Design Patterns for Scalable Apps
Reading Time: 9 minutes
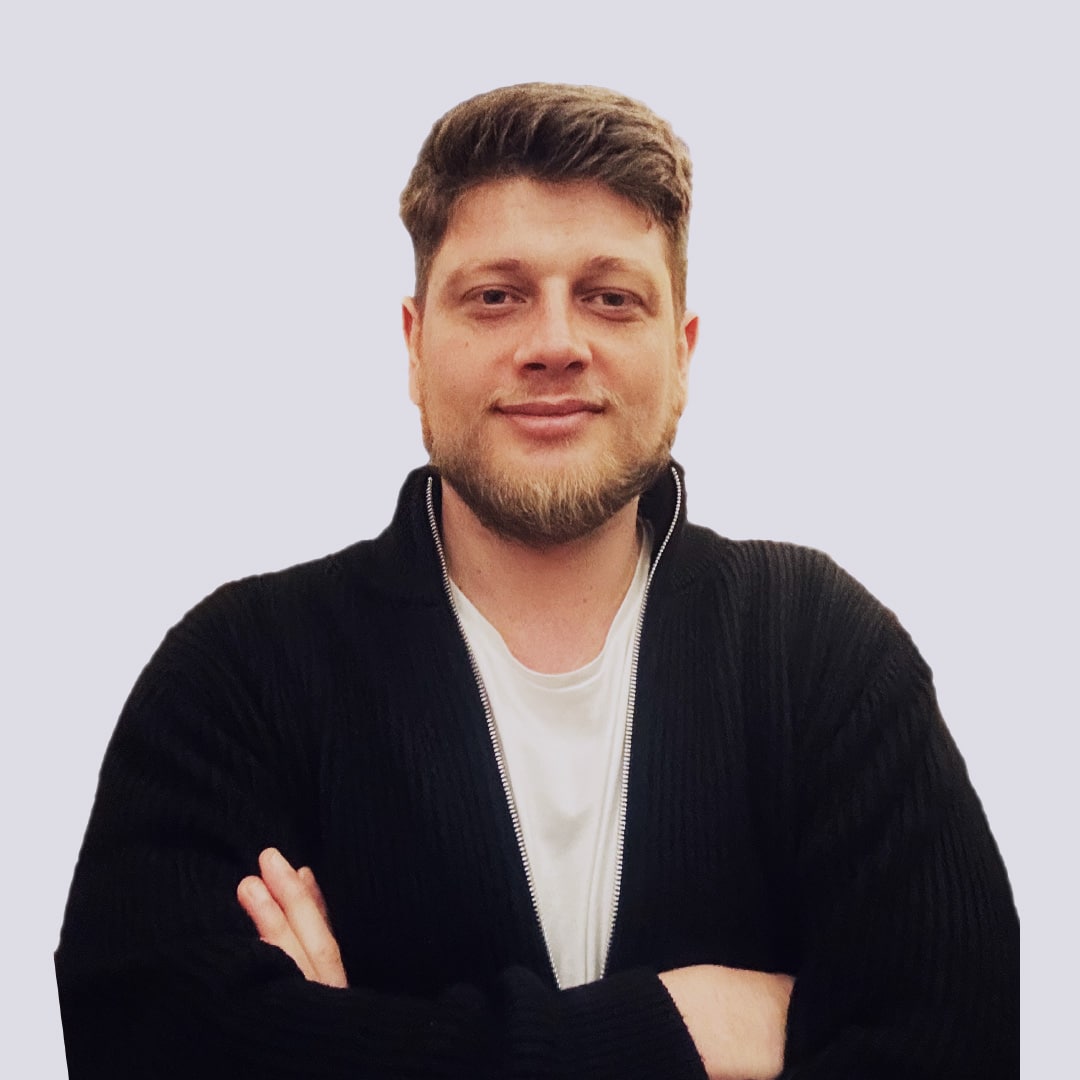
Kerem Karadeniz
Software Engineer
React has revolutionized the way developers build user interfaces, becoming the cornerstone of modern web development. Known for its flexibility, component-based structure, and declarative nature, React enables developers to create rich and dynamic user experiences. However, with this flexibility comes the challenge of maintaining a clean, organized, and scalable codebase. This is where design patterns come into play.
Design patterns in React are tried-and-tested solutions to common problems, offering a structured approach to writing code that is both reusable and maintainable. As your application grows in complexity, adopting these patterns can significantly enhance the overall development experience. They serve as blueprints, guiding developers toward building applications that are easy to understand, extend, and debug.
In this blog post, we’ll delve into five essential React design patterns that can elevate your development game:
- Render Props Pattern – A technique for sharing logic between components using a prop that is a function. This pattern emphasizes flexibility and composability, enabling components to dynamically decide how to render content.
- Container/Presentational Pattern – A foundational approach to separating concerns by dividing components into two categories: containers (handling logic and state) and presentational components (focusing solely on rendering).
- Custom Hooks Pattern – Introduced in React 16.8, hooks allow developers to encapsulate and reuse stateful logic in a functional component paradigm, streamlining code and reducing boilerplate.
- Higher-Order Component (HOC) Pattern – A pattern that leverages JavaScript functions to enhance components by wrapping them in higher-order functions, enabling reusability and separation of concerns.
- Compound Components Pattern – A pattern that allows components to work together seamlessly by using a parent component as the orchestrator, promoting intuitive and flexible APIs.
Why Design Patterns Matter in React
React’s core philosophy revolves around building reusable, modular components. While this is a powerful approach, it can sometimes lead to unstructured and hard-to-manage codebases, especially in larger applications. Design patterns play a critical role in mitigating these challenges by:
- Promoting Reusability: Design patterns help abstract common logic into reusable units, reducing redundancy and improving development speed.
- Improve Readability: A consistent use of patterns makes the codebase more intuitive, allowing developers to quickly grasp its structure.
- Enhancing Scalability: As applications grow, maintaining a clear separation of concerns and structured logic ensures that the code remains manageable and easy to extend.
- Encouraging Best Practices: By following established patterns, developers can avoid anti-patterns and pitfalls, leading to more robust and reliable applications.
Each design pattern serves specific use cases, addressing unique challenges in React development. For example, the Render Props Pattern is ideal for scenarios requiring dynamic rendering logic, while the Hooks Pattern is perfect for managing state and side effects in functional components.
Setting the Stage for Scalable Development
Before diving into the patterns themselves, it’s crucial to understand that there is no one-size-fits-all solution to software development. The choice of pattern depends on the context of the problem, the team’s familiarity with React, and the long-term goals of the application. By mastering these patterns, you’ll not only improve the quality of your code but also make your applications more maintainable and future-proof.
In the sections that follow, we will explore each of these patterns in detail. We’ll discuss their principles, implementation, advantages, and trade-offs, providing practical examples to help you apply them effectively in your projects. Whether you are a seasoned React developer or just embarking on your journey, understanding these patterns will be invaluable in building scalable, high-quality applications.
Let’s dive in and unlock the power of React design patterns!
1. Compound Component Pattern:
The Compound Components Pattern is a design pattern that allows components to collaborate seamlessly. This pattern is particularly effective in scenarios where components need to share context or logic but remain decoupled. By structuring components hierarchically and enabling a parent component to manage the shared state, the pattern simplifies the development of flexible and reusable APIs.
Implementation in React Native
Here’s an example of an Accordion component implemented using the Compound Components Pattern:
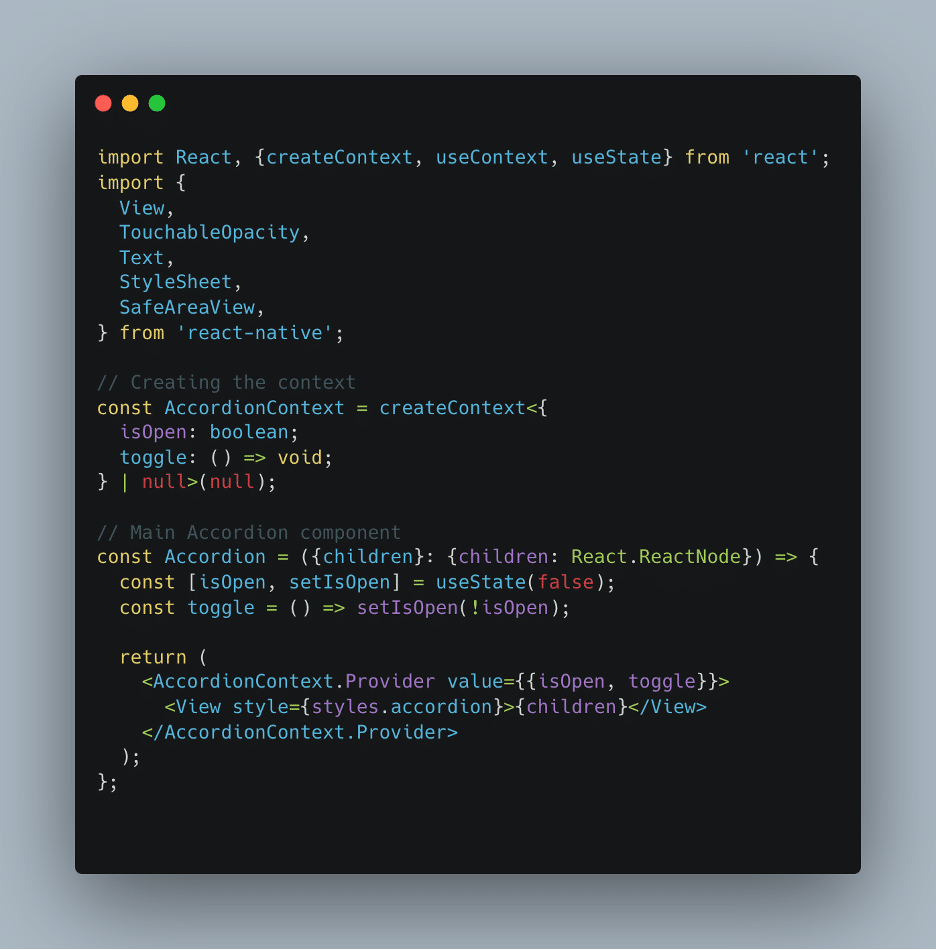
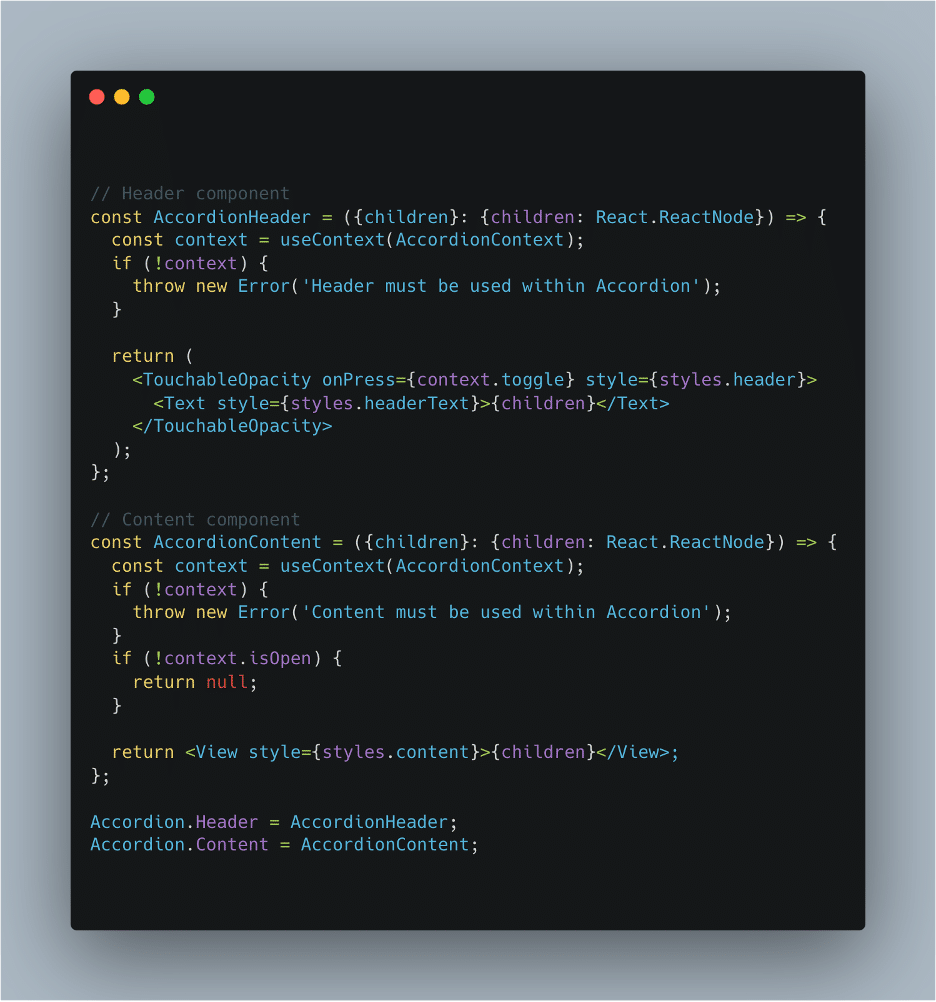
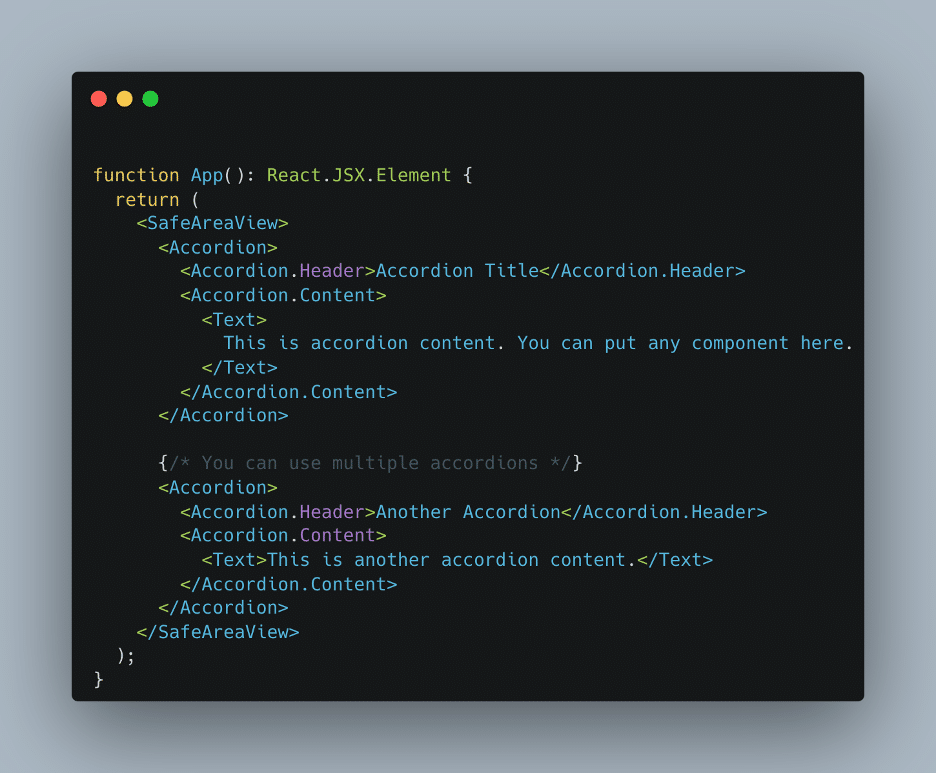
Advantages
- Intuitive API:
- The parent-child relationship allows for clear and predictable interactions between components.
- Decoupling Logic:
- Individual child components remain reusable and independent of one another.
- Improved Maintainability:
- Shared logic is encapsulated in the parent component, reducing duplication and improving consistency.
- Enhanced Readability:
- Hierarchical structure mirrors the DOM tree, making it easier to understand and manage.
Disadvantages
- Boilerplate Code:
- Implementing the pattern can introduce additional setup and increase code complexity.
- Limited Reusability of the Context:
- The pattern depends on the context provider, which may limit its reuse outside the component tree.
- Potential Overhead:
- Managing shared state for compound components can introduce unnecessary overhead for simpler use cases.
- The Steeper Learning Curve:
- Developers unfamiliar with context or compound patterns may find it challenging to implement initially.
The Compound Components Pattern is especially valuable in the React ecosystem’s for building modular and cohesive components. By structuring your code with this pattern, you can create a consistent and maintainable architecture for your applications.
2. Higher-Order Component (HOC) Pattern:
The Higher-Order Component (HOC) Pattern is a powerful design pattern in React and React Native, used to share functionality between components. HOCs are JavaScript functions that take a component as an argument and return a new component, adding additional functionality or behavior to the original component. This pattern is widely adopted for tasks like authorization, logging, theming, and data fetching.
Implementation in React Native
Here’s an example of an HOC that adds loading functionality to any component:
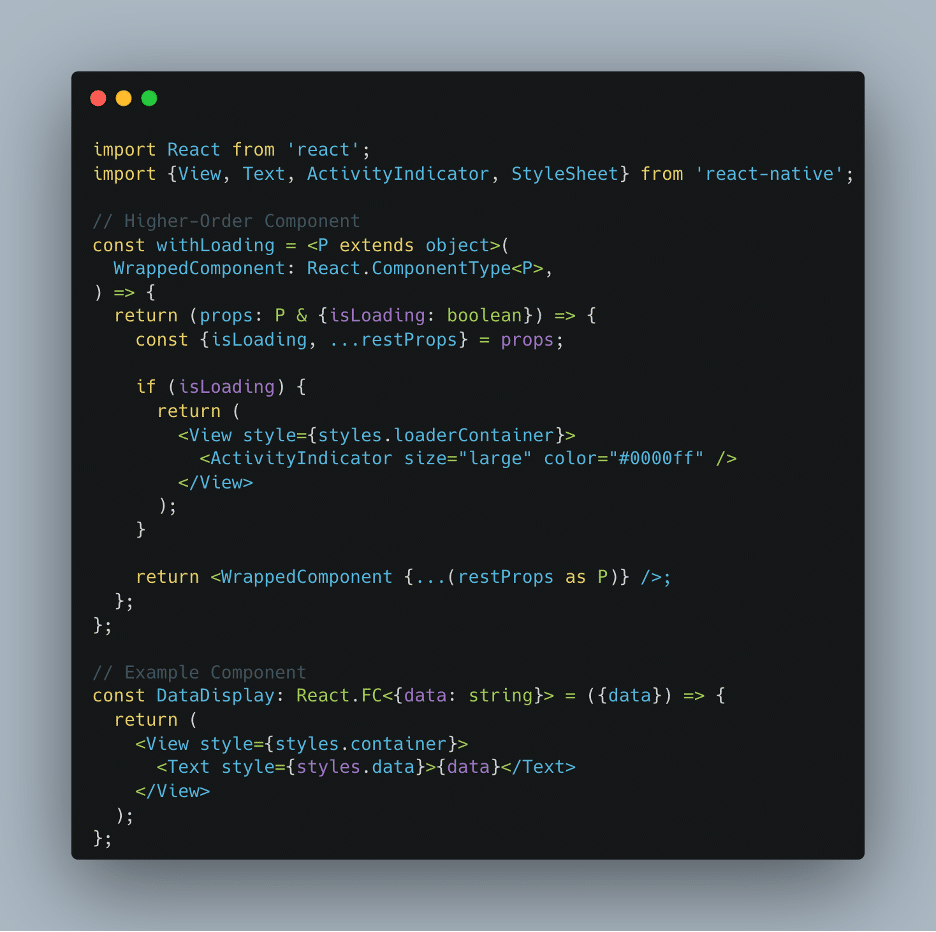
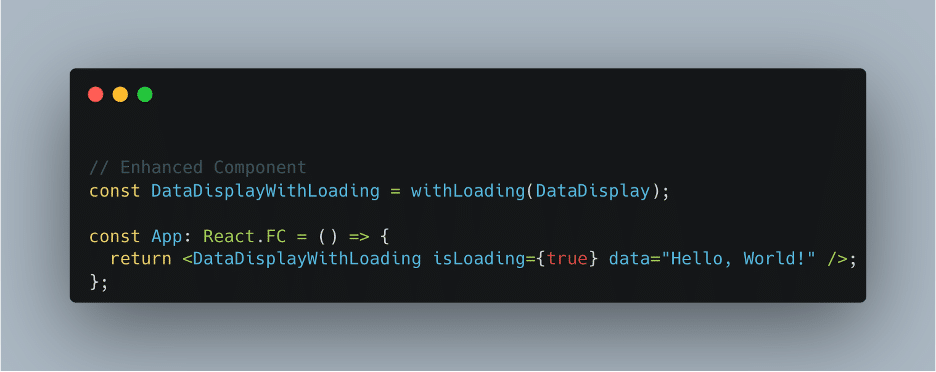
Advantages
- Code Reusability:
- HOCs allow you to reuse logic across multiple components, reducing duplication.
- Separation of Concerns:
- Functionality is abstracted into HOCs, making components simpler and focused on their primary tasks.
- Dynamic Enhancements:
- You can enhance components dynamically without modifying their original implementation.
- Composability:
- HOCs can be composed, allowing multiple functionalities to be added to a component in a structured way.
Disadvantages
- Increased Complexity:
- Wrapping components with multiple HOCs can lead to deeply nested structures, making the code harder to read and debug.
- Loss of Intuitive Props:
- Props passed to the HOC might be obscured, requiring developers to carefully manage prop forwarding.
- Reduced Performance:
- Each HOC adds an additional layer to the component tree, potentially impacting performance in complex applications.
- Static Method Loss:
- Static methods of the original component are not accessible in the HOC-wrapped component unless explicitly copied.
Use Cases for HOCs in React Native
- Authentication: Wrapping components to handle user authentication logic.
- Theming: Added dynamic theming capabilities to components.
- Data Fetching: Abstracting data-fetching logic to enhance components.
Despite these challenges, the High-Order Component Pattern remains a valuable tool for React developers. When used thoughtfully, HOCs can simplify complex logic, promote reusability, and keep components clean and maintainable.
3. Container/Presentational Pattern:
The Container/Presentational Pattern is a widely adopted approach in React applications, and its principles translate effectively to the React ecosystem. This pattern helps in organizing components by separating them into two distinct categories:
- Container Components:
- Handle the application’s state and logic.
- Interact with APIs, Redux stores, or other data sources.
- Pass data and callbacks as props to presentational components.
- Presentational Components:
- Focus solely on how things look.
- Render the UI based on props received from container components.
- Are typically stateless and functional components.
Implementation in React Native
Let’s consider an example of a user profile screen in a React Native application:
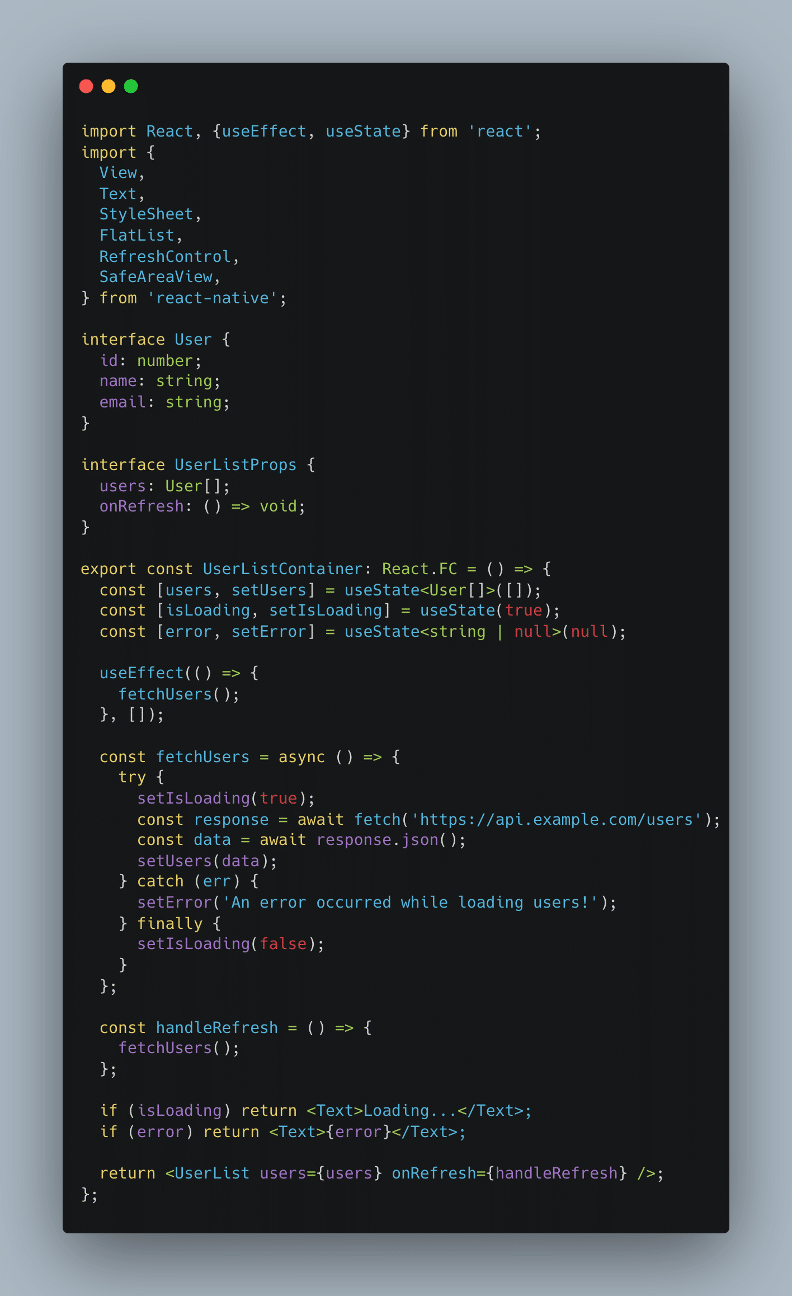
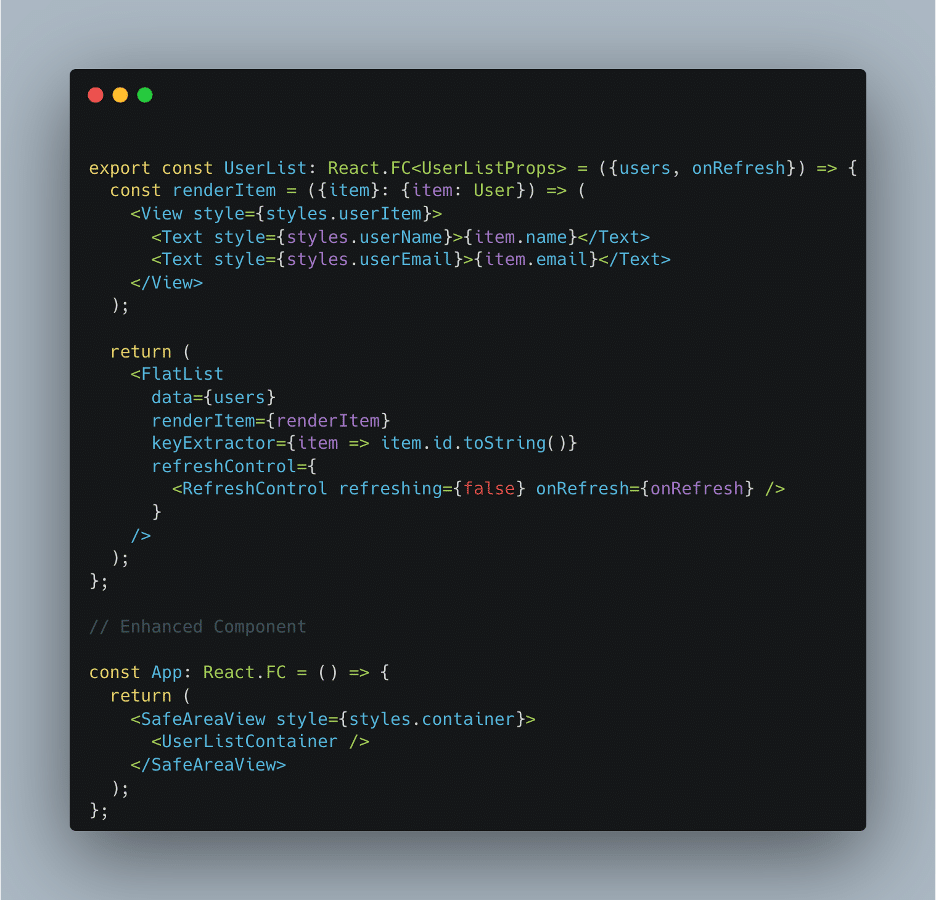
Advantages
- Separation of Concerns:
- Logic and presentation are neatly decoupled, making the components easier to understand and test.
- Presentational components can be reused in different contexts without modification.
- Improved Readability:
- Code is more organized, as container components handle the heavy lifting, while presentational components focus on rendering.
- Enhanced Reusability:
- Presentational components are more generic and can be used with different data sources or container components.
- Easy Testing:
- Presentational components are stateless and can be tested in isolation, while container components can focus on logic tests.
Disadvantages
- Boilerplate Code:
- Splitting logic and presentation can lead to an increase in the number of files and components, which might feel verbose for smaller projects.
- Prop Drilling:
- Passing props from containers to presentational components can become cumbersome, especially as the component hierarchy grows.
- Complex State Management:
- In larger applications, managing state and data flow exclusively through container components can lead to inefficiencies. State management libraries like Redux or Context API are often needed to mitigate this issue.
Despite these challenges, the Container/Presentational Pattern remains a highly effective strategy for building React applications with a clear and maintainable structure. By leveraging this pattern thoughtfully, developers can achieve a balance between clarity and complexity, ensuring that their codebases scale gracefully with the application.
4. Render Props Pattern:
The Render Props Pattern is another versatile and powerful design pattern used in React and React Native to share logic between components. In this pattern, a component accepts a function as a prop, and this function is used to determine what to render. This approach enables developers to dynamically control how a component behaves or renders, making it an excellent tool for handling reusable logic.
Implementation in React Native
Here’s an example of implementing the Render Props Pattern for a NetworkStatus component that checks internet connectivity:
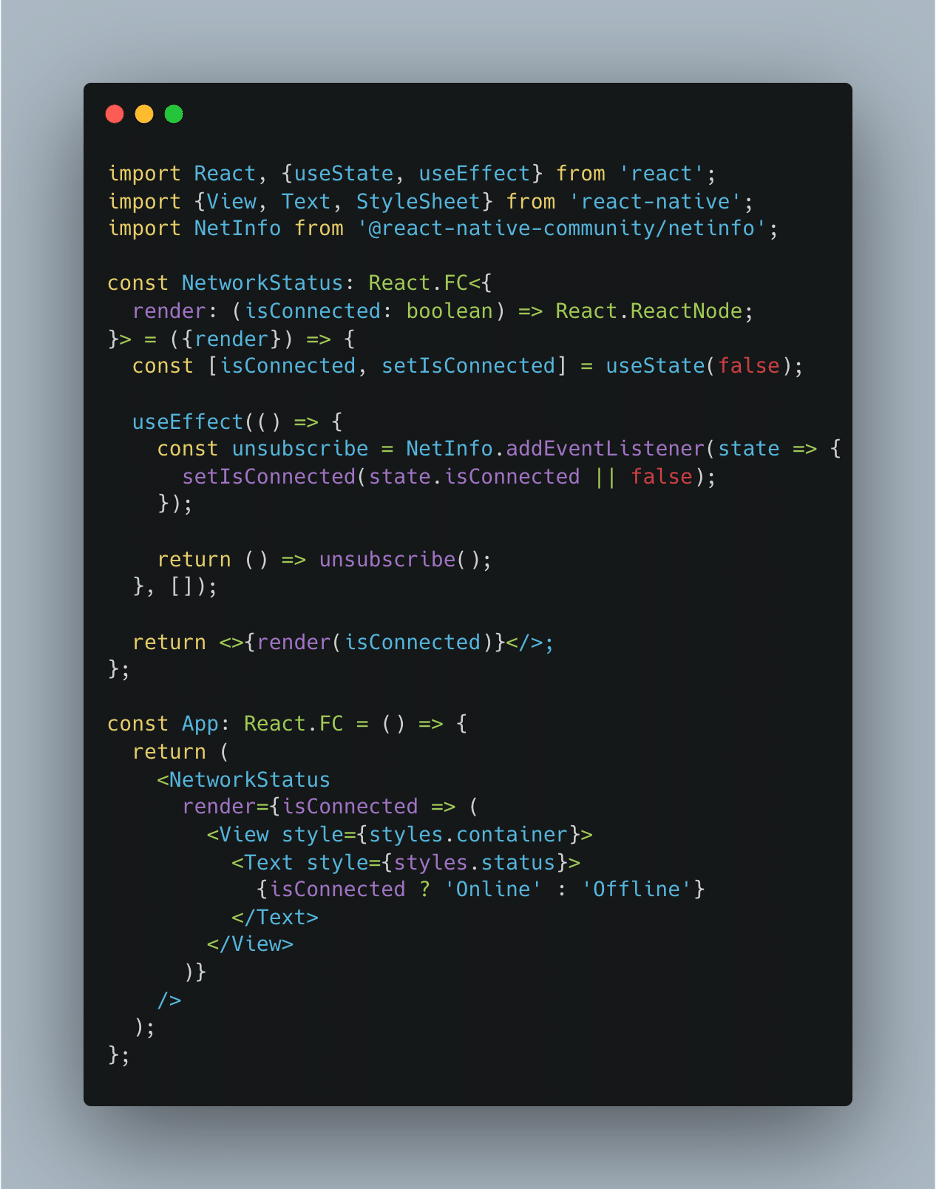
Advantages
- Reusability of Logic:
- Encapsulates shared behavior in one place, making it reusable across multiple components.
- Flexibility:
- Allows components to decide dynamically how shared logic should be rendered.
- Improved Separation of Concerns:
- Keeps logic and UI concerns separate, allowing for cleaner and more maintainable code.
- Dynamic Behavior:
- The same logic can be reused in different ways by passing different render functions.
Disadvantages
- Verbosity:
- Using render props can sometimes lead to nested and verbose code, especially when combined with other patterns.
- Performance Concerns:
- Passing functions as props can lead to unnecessary re-renders if not handled carefully.
- Complexity for New Developers:
- The pattern can be hard to grasp for those new to React or unfamiliar with functional programming paradigms.
Despite these drawbacks, the Render Props Pattern is an excellent choice for handling complex, reusable logic in React applications. By carefully structuring the render functions and minimizing unnecessary re-renders, developers can make the most of this pattern to build highly dynamic and reusable components.
5. Custom Hook Pattern:
The Custom Hooks Pattern is a powerful design strategy in React and React Native that leverages the flexibility of hooks to create reusable, modular logic. Custom hooks encapsulate logic into functional units, promoting reuse and reducing redundancy across your application.
Implementation in React Native
Here’s an example of a custom hook for managing network status:
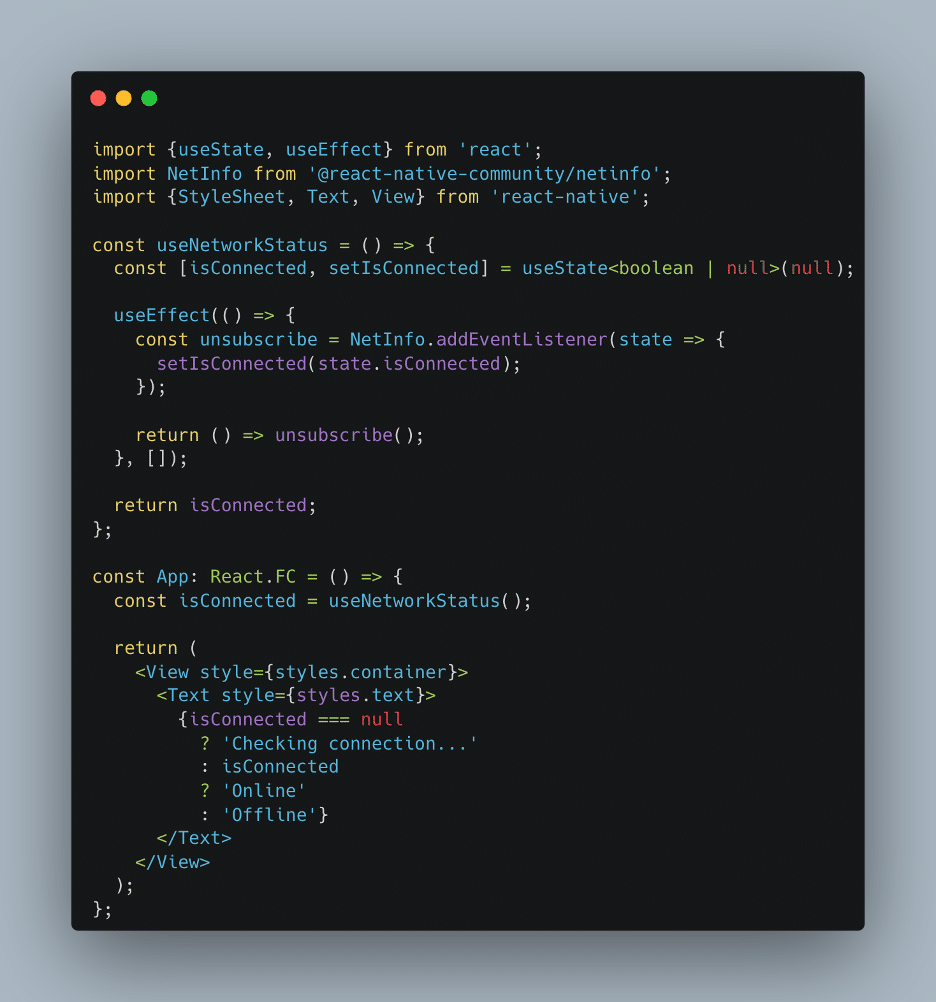
Advantages
- Reusability:
- Encapsulating logic in custom hooks allows you to reuse it across multiple components, reducing duplication.
- Improved Readability:
- Logic is separated from component rendering, making components cleaner and easier to read.
- Simplified Testing:
- Custom hooks can be tested independently, ensuring their behavior is predictable.
- Composability:
- Custom hooks can be combined to handle complex logic in a modular way.
Disadvantages
- Potential for Overuse:
- Creating overly specific custom hooks can lead to a fragmented codebase.
- Hidden Dependencies:
- Hooks may depend on external libraries or APIs, which might not be obvious at first glance.
- Debugging Complexity:
- Debugging hooks can be challenging, especially when dealing with stale closures or dependency issues in useEffect.
- Learning Curve:
- Developers new to hooks might find it difficult to understand or implement custom hooks effectively.
The Custom Hooks Pattern is an indispensable tool in React, enabling developers to write clean, reusable, and modular code. By using this pattern thoughtfully, you can significantly enhance the maintainability and scalability of your applications.
Key Takeaways
Design patterns are the cornerstone of clean, maintainable, and scalable software development. In the React ecosystem, they serve as a compass, guiding developers through the complexities of modern application design. By understanding and leveraging patterns like Render Props, Container/Presentational Components, Custom Hooks, Higher-Order Components, and Compound Components, we unlock the ability to build sophisticated applications that stand the test of time.
The true power of React lies in its flexibility and extensibility. However, this flexibility also comes with the responsibility of crafting a codebase that is robust and consistent. Design patterns help ensure that your projects remain scalable, readable, and easy to maintain, even as they grow in complexity. They encourage separation of concerns, promote reusability, and make it easier for teams to collaborate effectively.
As React continues to evolve and expand, the need for solid architectural practices becomes even more critical. The patterns discussed in this blog not only address common challenges in the React ecosystem but also pave the way for innovation and creativity. They empower developers to focus on solving business problems while maintaining high-quality code.
But design patterns are not just about following established rules; they are about understanding the “why” behind the rules. They are tools that must be applied thoughtfully, with consideration for the unique needs of each project. The choice of pattern often depends on the context, team dynamics, and project goals. Experimentation, critical thinking, and continuous learning are essential to mastering these patterns and adapting them to new challenges.
React thrives because of its active and passionate community. Sharing experiences, discussing architectural approaches, and learning from one another are what drive the ecosystem forward. By adopting and refining design patterns, you contribute to this shared journey of growth and innovation.
Design patterns in React are more than just technical solutions; they are a mindset—a way of thinking about building applications that are not only functional but also elegant and maintainable. As you move forward in your development journey, remember that the best code is not just written; it is designed. With the right patterns, a clear vision, and a commitment to excellence, the possibilities are limitless.
Thank you for exploring this deep dive into React design patterns. May these insights inspire you to craft applications that are as resilient and scalable as they are user-friendly. Here’s to a future of clean, efficient, and beautiful code in the ever-evolving React ecosystem!
Reading Time: 9 minutes
Don’t miss out the latestCommencis Thoughts and News.
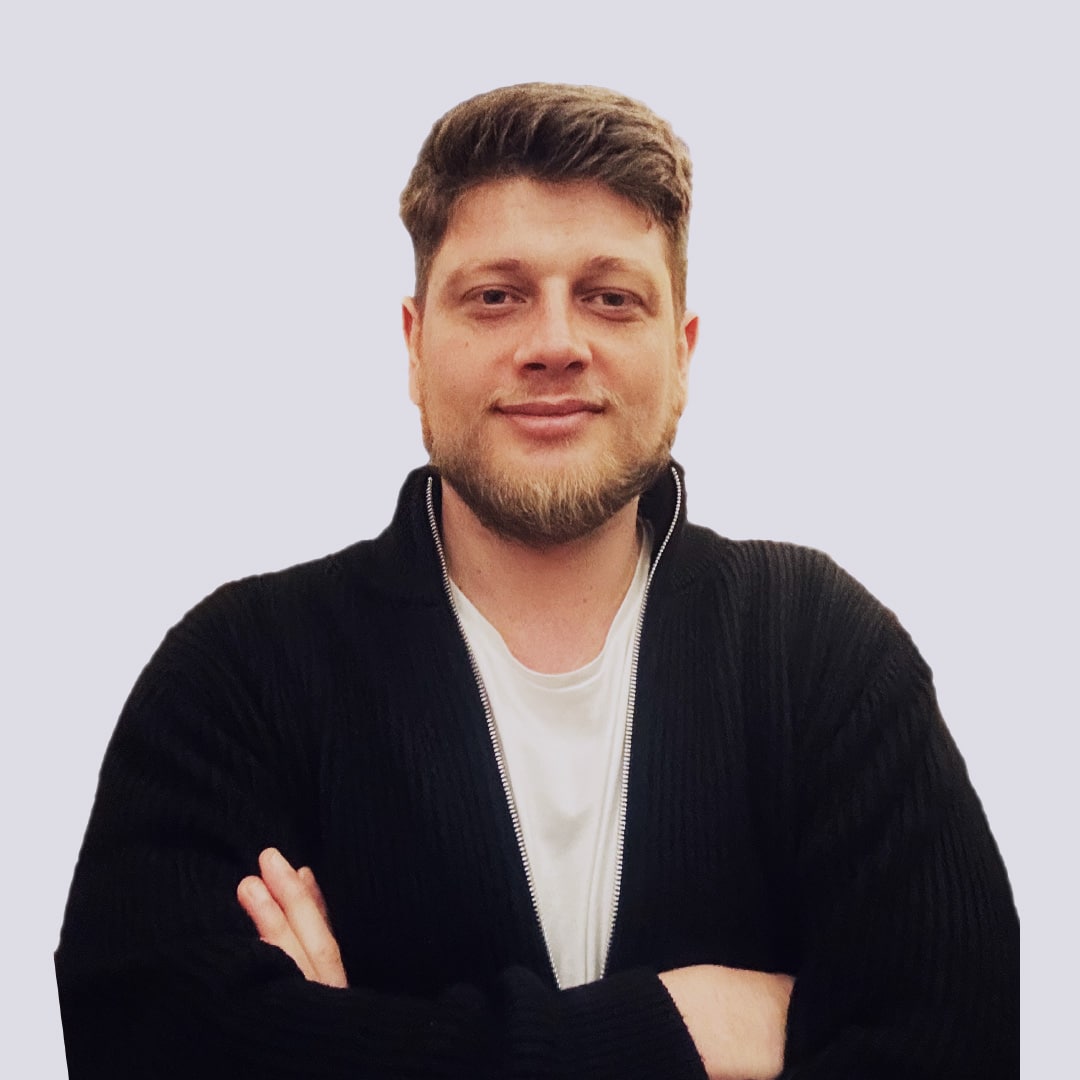
Kerem Karadeniz
Software Engineer